SpringBoot + MinIO实现文件切片极速上传技术
本文将介绍如何使用Spring Boot和MinIO实现文件切片极速上传技术,通过将大文件分割成小片段并并行上传,显著提高文件上传速度。
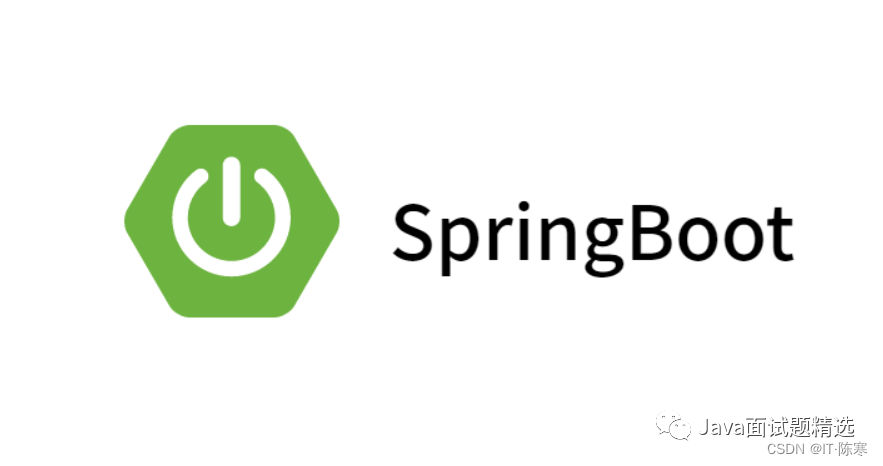
2文件切片上传简介
3技术选型
3.1 Spring Boot
3.2 MinIO
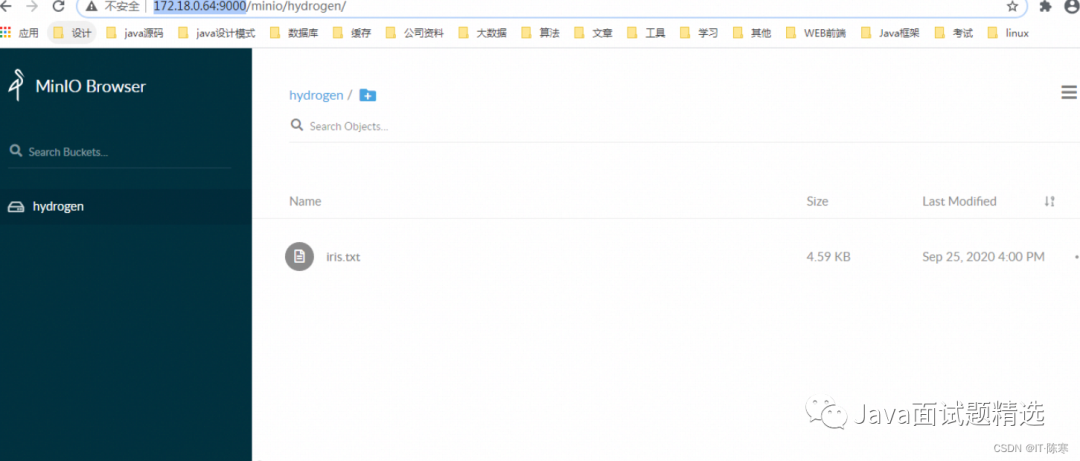
4搭建Spring Boot项目
<!– pom.xml –>
<dependencies>
<!– Spring Boot Web –>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!– Thymeleaf模板引擎 –>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!– MinIO Java客户端 –>
<dependency>
<groupId>io.minio</groupId>
<artifactId>minio</artifactId>
<version>8.3.3</version>
</dependency>
</dependencies>
5集成MinIO
5.1 配置MinIO连接信息
# application.properties
# MinIO配置
minio.endpoint=http://localhost:9000
minio.accessKey=minioadmin
minio.secretKey=minioadmin
minio.bucketName=mybucket
5.2 MinIO配置类
import io.minio.MinioClient;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MinioConfig {
@Value(“${minio.endpoint}”)
private String endpoint;
@Value(“${minio.accessKey}”)
private String accessKey;
@Value(“${minio.secretKey}”)
private String secretKey;
@Bean
public MinioClient minioClient() {
return MinioClient.builder()
.endpoint(endpoint)
.credentials(accessKey, secretKey)
.build();
}
}
6文件切片上传实现
6.1 控制器层
import io.minio.MinioClient;
import io.minio.errors.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
@RestController
@RequestMapping(“/file”)
public class FileController {
@Autowired
private MinioClient minioClient;
@Value(“${minio.bucketName}”)
private String bucketName;
@PostMapping(“/upload”)
public String upload(@RequestParam(“file”) MultipartFile file) {
// 实现文件切片上传逻辑
// …
return “Upload success!”;
}
}
6.2 服务层
import io.minio.MinioClient;
import io.minio.errors.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
@Service
public class FileService {
@Autowired
private MinioClient minioClient;
@Value(“${minio.bucketName}”)
private String bucketName;
public void uploadFile(String objectName, MultipartFile file) throws IOException, InvalidKeyException, NoSuchAlgorithmException, InsufficientDataException, NoResponseException, ErrorResponseException, InternalException, InvalidBucketNameException, XmlParserException, InvalidArgumentException {
// 实现文件切片上传逻辑
// …
}
}
6.3 文件切片上传逻辑
import io.minio.PutObjectArgs;
import io.minio.errors.*;
import java.io.IOException;
import java.io.InputStream;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
public class FileService {
// 省略其他代码…
public void uploadFile(String objectName, MultipartFile file) throws IOException, InvalidKeyException, NoSuchAlgorithmException, InsufficientDataException, NoResponseException, ErrorResponseException, InternalException, InvalidBucketNameException, XmlParserException, InvalidArgumentException {
InputStream inputStream = file.getInputStream();
long size = file.getSize();
long chunkSize = 5 * 1024 * 1024; // 每片大小5MB
long offset = 0;
while (offset < size) {
long currentChunkSize = Math.min(chunkSize, size – offset);
byte[] chunk = new byte[(int) currentChunkSize];
inputStream.read(chunk);
minioClient.putObject(
PutObjectArgs.builder()
.bucket(bucketName)
.object(objectName)
.stream(inputStream, currentChunkSize, -1)
.build()
);
offset += currentChunkSize;
}
inputStream.close();
}
}
7文件合并逻辑
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping(“/file”)
public class FileController {
// 省略其他代码…
@Autowired
private FileService fileService;
@PostMapping(“/merge”)
public String merge(@RequestParam String objectName) {
try {
fileService.mergeFile(objectName);
return “Merge success!”;
} catch (Exception e) {
e.printStackTrace();
return “Merge failed!”;
}
}
}
import io.minio.CopyObjectArgs;
import io.minio.GetObjectArgs;
import io.minio.PutObjectArgs;
import io.minio.errors.*;
import java.io.IOException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
public class FileService {
// 省略其他代码…
public void mergeFile(String objectName) throws IOException, InvalidKeyException, NoSuchAlgorithmException, InsufficientDataException, NoResponseException, ErrorResponseException, InternalException, InvalidBucketNameException, XmlParserException, InvalidArgumentException {
Iterable<io.minio.messages.Item> parts = minioClient.listObjects(bucketName, objectName);
// 通过CopyObject将所有分片合并成一个对象
for (io.minio.messages.Item part : parts) {
String partName = part.objectName();
minioClient.copyObject(
CopyObjectArgs.builder()
.source(bucketName, partName)
.destination(bucketName, objectName)
.build()
);
}
// 删除所有分片
for (io.minio.messages.Item part : parts) {
String partName = part.objectName();
minioClient.removeObject(bucketName, partName);
}
}
}
8页面展示
<!DOCTYPE html>
<html lang=”en” xmlns:th=”http://www.thymeleaf.org”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>File Upload</title>
</head>
<body>
<form id=”uploadForm” action=”/file/upload” method=”post” enctype=”multipart/form-data”>
<input type=”file” name=”file” id=”file” />
<input type=”submit” value=”Upload” />
</form>
<div id=”progress” style=”display: none;”>
<progress id=”progressBar” max=”100″ value=”0″></progress>
<span id=”percentage”>0%</span>
</div>
<script>
document.getElementById(‘uploadForm’).addEventListener(‘submit’, function (event) {
event.preventDefault();
var fileInput = document.getElementById(‘file’);
var file = fileInput.files[0];
if (!file) {
alert(‘Please choose a file.’);
return;
}
var formData = new FormData();
formData.append(‘file’, file);
var xhr = new XMLHttpRequest();
xhr.open(‘POST’, ‘/file/upload’, true);
xhr.upload.onprogress = function (e) {
if (e.lengthComputable) {
var percentage = Math.round((e.loaded / e.total) * 100);
document.getElementById(‘progressBar’).value = percentage;
document.getElementById(‘percentage’).innerText = percentage + ‘%’;
}
};
xhr.onload = function () {
document.getElementById(‘progress’).style.display = ‘none’;
alert(‘Upload success!’);
};
xhr.onerror = function () {
alert(‘Upload failed!’);
};
xhr.send(formData);
document.getElementById(‘progress’).style.display = ‘block’;
});
</script>
</body>
</html>
9性能优化与拓展
9.1 性能优化
-
并发上传:利用多线程或异步任务,将文件切片并行上传,提高上传效率。 -
分布式部署:将文件存储和应用部署在不同的服务器,减轻单个服务器的负担,提高整体性能。
9.2 拓展功能
-
断点续传:支持文件上传中断后的断点续传功能,提高用户体验。 -
权限控制:使用MinIO的访问策略进行权限控制,确保文件上传安全性。
10总结
微信赞赏
支付宝扫码领红包